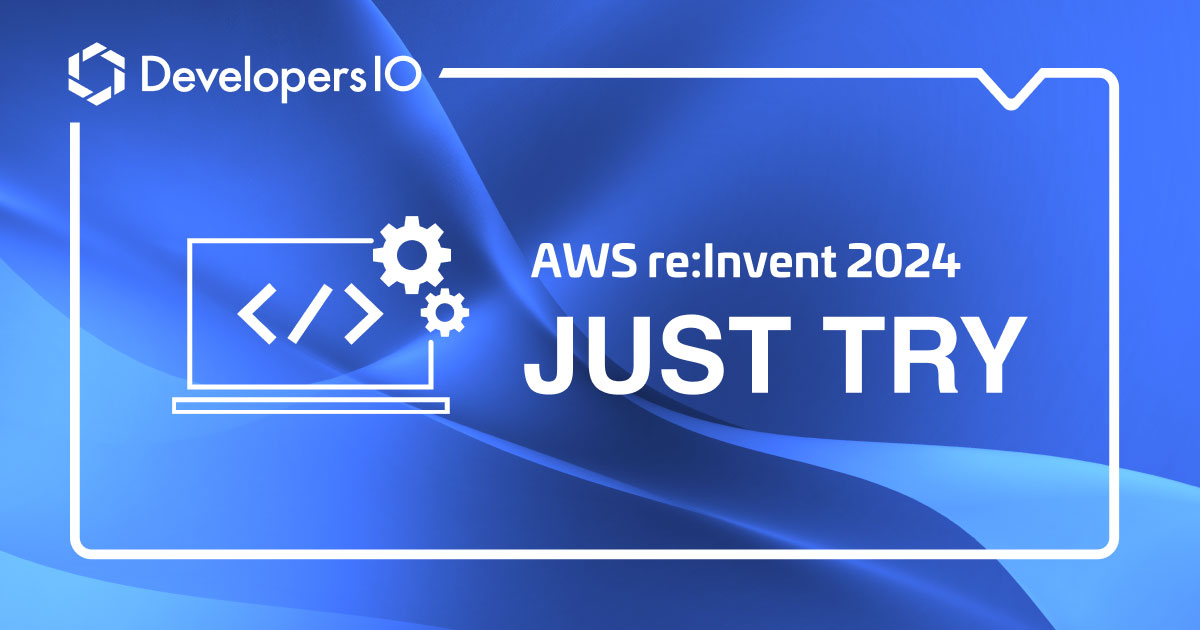
Let's Try AWS Console-to-Code
I learned about the AWS Console-to-Code service during re:Invent 2024 and decided to give it a try.
Hands-On
To test Console-to-Code, an EC2 instance was created using the following steps:
- Open the EC2 console
- Navigate to the top-right corner and click the Console-to-Code icon.
- Select Start recording.
With recording enabled, any actions performed in the AWS Management Console are captured, making it possible to generate CLI commands or Infrastructure-as-Code (IaC) templates for the created resources.
Next, launch an EC2 instance using the Launch Instance wizard. After the instance is successfully launched, return to the Console-to-Code menu and select Stop to end the recording.
The recording shows that three AWS resources were created. Select RunInstances and choose Copy CLI to retrieve the AWS CLI command for launching the instance:
aws ec2 run-instances --image-id "ami-0123456789xxxxxxx" --instance-type "t2.micro" --network-interfaces '{"AssociatePublicIpAddress":true,"DeviceIndex":0,"Groups":["sg-0ecf56e92f1e2a8da"]}' --credit-specification '{"CpuCredits":"standard"}' --tag-specifications '{"ResourceType":"instance","Tags":[{"Key":"Name","Value":"My Web Server"}]}' --metadata-options '{"HttpEndpoint":"enabled","HttpPutResponseHopLimit":2,"HttpTokens":"required"}' --private-dns-name-options '{"HostnameType":"ip-name","EnableResourceNameDnsARecord":true,"EnableResourceNameDnsAAAARecord":false}' --count "1"
Generating AWS CDK Python Code
To generate AWS CDK Python code, select Generate CDK Python.
To generate code, Console-to-Code requires your consent to make cross-Region calls within this AWS account. You can update cross-Region preferences in your Console-to-Code settings.
The CDK Python code is generated. You can customize it for your specific use case.
from aws_cdk import (
aws_ec2 as ec2,
Stack,
CfnOutput
)
from constructs import Construct
class MyStack(Stack):
def __init__(self, scope: Construct, construct_id: str, **kwargs) -> None:
super().__init__(scope, construct_id, **kwargs)
# Create a security group
security_group = ec2.SecurityGroup(
self, "WebServerSG",
vpc=ec2.Vpc.from_lookup(self, "VPC", is_default=True),
description="Allow HTTP traffic",
allow_all_outbound=True
)
security_group.add_ingress_rule(
ec2.Peer.any_ipv4(),
ec2.Port.tcp(80),
"Allow HTTP traffic"
)
# Create an EC2 instance
instance = ec2.Instance(
self, "WebServerInstance",
instance_type=ec2.InstanceType("t2.micro"),
machine_image=ec2.MachineImage.lookup(
name="ami-0123456789xxxxxxx"
),
vpc=ec2.Vpc.from_lookup(self, "VPC", is_default=True),
security_group=security_group,
instance_initiated_shutdown_behavior=ec2.InitiatedShutdownBehavior.STOP,
credit_specification=ec2.CreditSpecification(
cpu_credits=ec2.CpuCredits.STANDARD
),
metadata_options=ec2.InstanceMetadataOptions(
http_endpoint=ec2.InstanceMetadataEndpointService.ENABLED,
http_put_response_hop_limit=2,
http_tokens=ec2.InstanceMetadataHttpTokens.REQUIRED
),
private_dns_name_options=ec2.PrivateDnsNameOptions(
hostname_type=ec2.HostnameType.IP_NAME,
enable_resource_name_dns_a_record=True,
enable_resource_name_dns_aaaa_record=False
)
)
instance.instance.add_user_data(
"#!/bin/bash\n"
"yum install -y httpd\n"
"systemctl start httpd\n"
"systemctl enable httpd\n"
)
instance.instance.instance.add_tag("Name", "My Web Server")
CfnOutput(
self, "InstancePublicIp",
value=instance.instance_public_ip,
description="Public IP of EC2 instance",
export_name="InstancePublicIp"
)
# Reasoning: This code creates an EC2 instance with the specified configuration from the provided AWS CLI commands. It creates a security group that allows inbound HTTP traffic, and then creates an EC2 instance with the specified AMI, instance type, network interface, credit specification, tags, metadata options, and private DNS name options. The instance is configured to install and start the Apache HTTP server, and its public IP address is exported as a CloudFormation output. The code follows best practices for infrastructure as code by using the AWS CDK to define the resources in a declarative and reusable way.
Generating CloudFormation template in YAML format:
Similarly, select Generate CFN YAML to generate the CloudFormation template.
The CloudFormation template is generated.
Resources:
EC2Instance:
Type: AWS::EC2::Instance
Properties:
ImageId: ami-0123456789xxxxxxx
InstanceType: t2.micro
NetworkInterfaces:
- AssociatePublicIpAddress: true
DeviceIndex: 0
GroupSet:
- sg-0ecf56e92f1e2a8da
CreditSpecification:
CPUCredits: standard
Tags:
- Key: Name
Value: My Web Server
MetadataOptions:
HttpEndpoint: enabled
HttpPutResponseHopLimit: 2
HttpTokens: required
PrivateDNSNameOptions:
HostnameType: ip-name
EnableResourceNameDnsARecord: true
EnableResourceNameDnsAAAARecord: false
Reasoning: {The provided AWS CLI command runs an EC2 instance with the specified properties. The generated CloudFormation YAML code creates an EC2 instance resource with the same properties as specified in the CLI command. The ImageId, InstanceType, NetworkInterfaces, CreditSpecification, Tags, MetadataOptions, and PrivateDNSNameOptions properties are mapped directly from the CLI command to the CloudFormation resource properties. The count parameter is not included as CloudFormation creates a single resource by default.}
Preview code
In the previous steps, the console actions for launching an EC2 instance were recorded and converted into code. However, both the EC2 instance and Auto Scaling group launch wizards offer a Preview code feature.
This allows you to generate the code without actually creating the resources.
EC2 Launch Wizard
In the EC2 launch wizard, select Preview code located below the Launch instance button.
The Console-to-Code window appears, allowing the generated code to be reviewed.
Auto Scaling Group Launch Wizard
Similarly, in the Auto Scaling Group launch wizard, navigate to the Review page and select Preview code.
The Console-to-Code window appears, displaying the generated code for review.
Summary
AWS Console-to-Code was used to convert operations performed in the AWS Management Console into CLI commands, CDK code, and CloudFormation templates. Currently, this feature is available for services such as EC2, RDS, and VPC. Additionally, the preview code feature enables the generation of code without creating any resources.